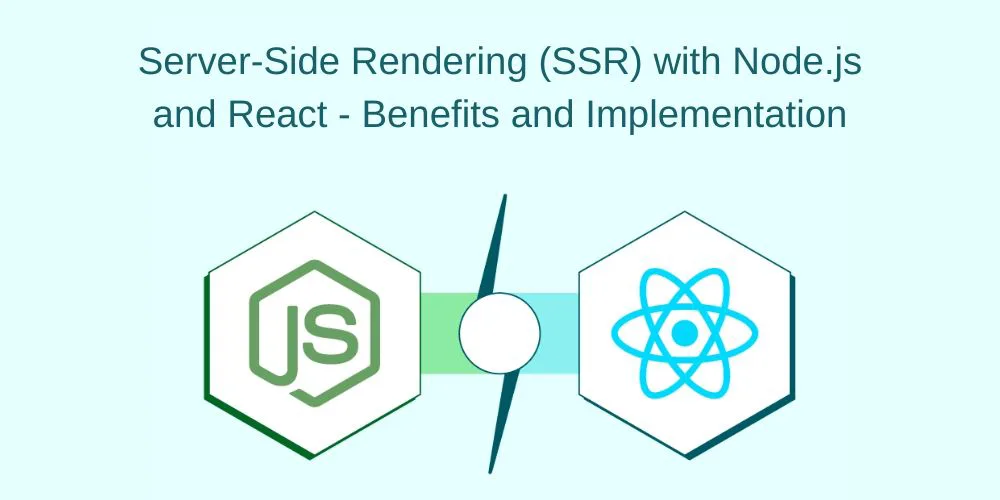
Delivering excellent user experiences while maintaining optimal speed has become crucial in the fast-paced world of web development. Due to this, Server-Side Rendering (SSR) approaches are now more often used, especially when utilising well-liked front-end frameworks like React.
In this article, written by NodeJS development Company for experts looking to improve their online applications, we'll discuss the advantages of SSR and walk you through its implementation using Node.js and React.
So, let’s get in!
Understanding Server-Side Rendering (SSR)
In contrast to the conventional method, where the browser gets raw JavaScript and renders the data, server-side rendering (SSR) includes creating HTML content on the server and transmitting it to the client.
This indicates that meaningful content can already be displayed on the first page to load from the server, even before JavaScript is fully loaded and processed.
Now, here are some of the benefits of Server-Side Rendering (SSR):
Improved Page Load Speed
SSR's effect on page load time is one of its main benefits. Users are able to access material more quickly because to the server transmitting pre-rendered HTML, which improves performance as a whole. For maintaining user interest and lowering bounce rates, this is very important.
Search Engine Optimization (SEO)
SEO improves immensely from SSR. Unlike single-page applications (SPAs), which frequently rely on JavaScript for content rendering, the content of pages rendered on the server is simple for search engines to crawl and index. This improves search engine visibility and increases the likelihood of ranking highly in search results.
Accessibility
SSR may have advantageous effects on accessibility. Users with impairments who rely on assistive technologies can access the information more successfully because it is present on the initial load.
Social Media Sharing
Pre-rendered content improves the user experience when sharing links on social media sites since it ensures that the shared link will show the right content sample and image.
Node.js: Empowering Server-Side Rendering
Node.js has emerged as a powerhouse in server-side technologies, making it an ideal choice for implementing Server-Side Rendering (SSR). Leveraging the V8 JavaScript engine, Node.js allows developers to run JavaScript on the server, providing a unified language for front and backend development.
Here we will explore how Node.js plays a pivotal role in SSR implementation.
1. Server Configuration with Express.js
Express.js, a minimalist web application framework for Node.js, simplifies setting up an SSR-enabled server. By utilising its intuitive routing and middleware capabilities, you can quickly create a server that handles rendering React components on the server side.
The server bridges your front-end application and the browser, efficiently delivering pre-rendered content for an enhanced user experience.
2. Package Management and Modules
Node.js's package management system, powered by npm (Node Package Manager), offers an extensive ecosystem of modules and packages that streamline development. This enables you to integrate third-party libraries and tools essential for SSR implementation easily.
You can efficiently manage dependencies with npm, ensuring a smooth development process and a robust SSR application.
React: Elevating User Experiences
React, a JavaScript library for building user interfaces has gained immense popularity for its component-based architecture and efficient rendering. Incorporating React into the SSR process enhances user experiences by providing meaningful content during the initial load, setting the stage for a seamless transition to interactive client-side behaviour.
Here, we will see how React synergises with SSR to elevate user experiences.
1. Component Reusability
React's component-centric approach aligns perfectly with SSR's goal of reusing components on both the server and client sides. Components encapsulate functionality and UI elements, allowing you to create consistent views rendered efficiently on both ends.
This reusability streamlines development and ensures a coherent user experience across devices and platforms.
2. Virtual DOM and Efficient Rendering
React's Virtual DOM mechanism, which represents the UI as an in-memory data structure, is crucial to SSR efficiency. When combined with server-side rendering, the Virtual DOM enables React to render components on the server, generate HTML content, and send it to the client.
This process minimises unnecessary browser rendering and contributes to faster initial page loads, enhancing the perception of speed for users.
3. Client-Side Hydration
While SSR provides the advantage of delivering pre-rendered content, React's client-side hydration ensures a seamless transition to interactive client-side behaviour. Hydrating the client-side application involves attaching event listeners and behaviours to the pre-rendered content, resulting in a fully interactive and dynamic user interface.
This combination of server-side rendering and client-side hydration creates a harmonious user experience.
Implementing SSR with Node.js and React – What’s the Procedure?
Implementing Server-Side Rendering (SSR) with Node.js and React opens up a world of possibilities for creating web applications with enhanced performance, improved search engine visibility, and better user experiences.
Let's dive in and explore the world of SSR implementation with these cutting-edge technologies.
Setting Up Your Project
Start by creating a new React application using your preferred project setup tool. You can use create-react-app or a custom Webpack configuration. Make sure to structure your components so that they can be rendered both on the server and the client.
Server-Side Rendering Logic
For implementing SSR, you'll need to create an Express.js server that will handle the rendering on the server side. First, install the required packages:
In this example, the App component is wrapped with StaticRouter to handle routing on the server side. The rendered content is then injected into the HTML template and sent to the client.
Adjusting Client-side Code
You need to adjust your client-side code to ensure a smooth transition from server-rendered content to client-side interactivity. Use the hydrate function from react-dom to attach event listeners and behaviours to the server-rendered content:
Building and Running Your Application
Build your React application using your preferred build tool, such as Webpack or Parcel:
npm run build
Now, start your Express server:
node server.js
Your SSR-enabled React application should now be accessible at http://localhost:3000.
To Conclude,
Server-side rendering with Node.js and React presents a powerful approach to enhancing web application performance, user experience, and search engine visibility. By pre-rendering content on the server, you can achieve faster initial page loads, improved SEO, and better accessibility.
Leveraging technologies like Express.js and React, the implementation process becomes more accessible than ever for professionals looking to optimise their applications for the modern web. Incorporating SSR into your development toolkit can ultimately lead to higher user satisfaction and increased engagement.