.png)
Introduction to Parking Lot Service:
- First, understand what the design actually is and what are all the requirements before directly jumping into designing the parking lot system. Since the parking lot system design is a very vague question so, do not assume anything on your own.
- You have to concentrate on your system design and especially object-oriented design skills. How well you can design the whole system in terms of components and classes and how will your model parking-lot system into different classes and objects identify constraints and design interfaces. You have to be well versed in object-oriented design concepts like abstraction, encapsulation, inheritance, and polymorphism. You have to define the links and associations between the classes and objects in your system.
Another thing that you need to consider is that when you are designing a parking-lot system.
Requirement Gathering:
Let's see, Java development services company consider that we have a very huge parking lot if you google you will find that there are many parking lots with parking sizes of more than 5,000 or even more. For example, the largest parking lot is in West Edmonton in Canada which has more than 15,000 parking spots for vehicles. So, we are talking about parking spaces in the range of 5,000 to 15,000.
Functional Requirements:
1. Parking Spots:
- So, the first requirement is parking lots 5000 to 15k spots. These huge parking lots will have more than one entrances and exits. In our case let's assume that our parking lot has two entrances and two exits.
2. Provisioning of Ticket to Customer:
- The Second requirement is the customers collect tickets at the entrances and the parking spot is assigned on the ticket that which parking space is being allotted to that customer mentioned in that coupon.
3. Short distance Parking Sport allocation
- The third requirement is the parking spot allotted to a vehicle should be near to the entrance area and it means that if a customer is entering into the parking lot from this entrance then the parking area which got assigned should be the nearest from this entrance and if another customer is actually entering the parking lot from some other entrances then the parking spot assigned to that customer the nearest form another entrance as we have total two entrances.
4. Maintaining the Capacity of Parking lot. (In our case max limit is 15k vehicles)
- The Fourth comment is the system should not allow more vehicles then the maximum capacity of the parking lot. So, there is a limit or capacity and we can assume simply that the capacity that we have in our parking lot system say 15,000. So, after 15k we should not allow any other customer.
5. Categorizing the Parking Spot
- The Fifth requirement is that there will be different types of a parking spots for example there will be parking spots for handicapped people or ambulance and another for an emergency parking spot. There will be parking spots for compact cars and other for large cars etc. So, in our design we will consider there are five types of parking spots. And these will be handicapped parking, compact car, Emergency parking spot, large vehicle and bike parking spot.
6. Parking Fee
- The sixth requirement is the system should support an hourly parking rate and the final parking fee should be based on the total time that a vehicle is spent in the parking lot. The customers can pay parking fee both with cash and credit card or google pay(UPI).
7. Parking surveillance system
- There should be a monitoring system as well. The monitoring system would be responsible for monitoring how many cars are entering and exiting etc. and of course there could be video surveillance as well as part of the monitoring system.
8. Design Flexibility & Code Reusability
- Now the last requirement is to design the system in such a way so that it can be applied for this toleration of parking system for more and more parking lots with minimal changes in the code and design. This means you can take your system and apply it to a different parking lot maybe for a different shopping mall parking lot or an airport parking lot with minimal changes mostly due to the configurations as of that new parking lot describing the overall structure of the parking lot, the number of flows the capacity and the different types of parking spots.
So what you would like to do is you would like to have your one system designed and then you what you would like to do is that you just want to apply your system that you have designed and you want to reuse that system for the installation of different parking lot systems and the only thing that you would like to now change is the configuration information.
Scaling the Requirement if needed:
- An Increasing number of Parking slots from 5k to 30K
- Increase in data Storage due to multiple slots extension
- Increasing in data storage due to increase in the number of tickets per day (May be per day 1 MB)
- Maintaining parking lot data for 5 years at least
⮚ Here is my example, I am just simplifying the requirements by coming up with a simple design. We will design that parking lot system using some design patterns. There are three broad categories of design patterns the first one is Creational design patterns Creational design patterns deals with how the objects are instantiated. The second type of design patterns structural design patterns.
⮚ The structural design patterns discuss how different objects and classes are composed in order to form a larger structure and the third design patterns are behavioral design patterns. The behavioral design patterns deal with these possibilities of the objects and how they interact with each other. Before going into the design of the parking lot we are also going to discuss the design approach that we will be taking to design the parking lot system.
Parking Lot Design Approach:
Top-Down Approach
- So, there are two different design approaches that people usually take the first one is top-down design and the second one is the bottom-up in the top-down design we first construct the high-level object and then we identify these smaller subcomponents in it and then we design those subcomponents and then in each sub-component we identify its sub-components and then we had then design those sub-components.
⮚ Whereas in a bottom-up approach we first design the smallest component and then we use those smallest components to design a bigger component and so on. Please note that the bottom-up approach is aligned with object-oriented design and so we should be using the bottom-up design approach while designing a Parking lot system. At this point we should identify different objects and actions in our system.
- Parking Lot System
- Entry/Exit terminals
- Exit Payment Processor
- Parking Spot
- Coupon issuing
- Surveillance system
So please understand the difference between a vehicle parking spot and a vehicle class. The parking lot system will be using the vehicle parking spot, but it will not be using a vehicle class. As there is no reason to use a vehicle class along with its subclasses.
Bottom-up Approach:
- Now let's design different interfaces classes and components in our parking lot system using bottom-up approach. So a vehicle parking spot is an object in our system there could be different types of hiding spot example we could have a parking spot for the handicapped, we could have a compact and large parking spot we could have a emergency parking spot and also we could have motorcycle parking spot as we have discussed in the requirements.
- Now there are two different ways to represent those different types of parking spots. We can use ENUM types to represent each type of parking spot however this shows that you are not that proficient in the object oriented design as this approach has some flaws for example if you need to add another parking spot type then it could require changes at various places in the code which would violate the open/close design principle this principle dictates that existing and well tested classes should not be modified whether new feature needs to be built.
- The most preferable approach is that we actually drive each different types of parking spot from the apparent parking spot interface or class. So, this diagram shows all the different types of parking spot available in our system in terms of different classes.
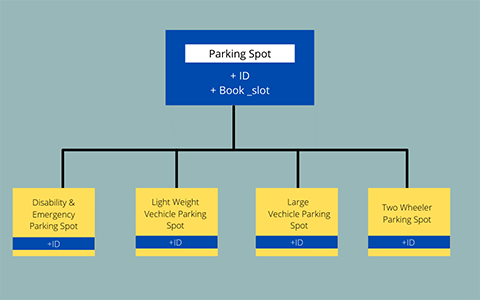
Explanation:
- So we have a parent parking spot class and which has an ID and deserves as property and there will be methods to get the property like getID() and there would be a getter method to get the properties as well. Now if you see we have handicapped parking spots, compact parking spots, Emergency Parking spots, large parking spots, and bike spots. Six spots are different classes which are derived from this parking spot and now in that case, if we have to add another type of parking spot what we would need to do is just actually extend this parking spot class by some other parking spot class. Here, we have to make the parking spot class as an abstract class, what it means is that we cannot instantiate an object of a parking spot.
- We could instantiate an object of a handicapped parking spot or large or compact parking spot but not the parent parking spot class object. We will have a parking Coupon where we will have ticket ID, parking spot ID and the ticket will contain information about this sport or the parking slot number where the car needs to be parked then the parking spot type and the coupon issued time which is the time when the vehicle enters the parking lot.
- There are two types of terminals that we have in our parking lot system which are the entry terminal and exit terminal and so we will have a terminal class which would have a getId() as a class getter function and then from this terminal class we would actually drive two different classes one for the entry terminal and other for the exit terminal and you can see right now the entry terminal have getParkingTicket()/ getParkingCoupon()with a parking spot type and then we have an exit terminal which accepts the coupon with the method acceptParkingCoupon() & acceptParkingTicket() and it takes a parking coupon as input as shown below
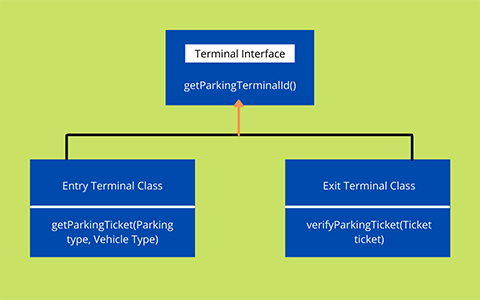
⮚ So, these are classes or interfaces which will be implemented by the actual class object.
⮚ Now the next set of interfaces and classes that I am mentioning to the assignment of parking spot to a vehicle so we will have an interface called parking assignment strategy it will have a getParkingSpot()method which will take a Parking Terminal as an input.
⮚ This term that is the entry terminal. The getParkingSpot() will return a parking spot and then it will also have a release parking spot which will take a parking spot as input and of course it will mark that parking spot as free or available again and now we will be using the strategy design pattern where we actually inherit a parking spot near Entrance strategy class which will implement the algorithm to actually figure out the nearest parking spot and they turn that parking spot to the entering customer.
How to find out the nearest Parking Spot availability
- So, here we have to deal with the assignment of the parking spot. The best way to implement it using min heaps. So, we will have a number of min heaps as the number of entrances and all the parking spots will be added to all those min heaps. For each entry terminal there will be a min heap this so we will have 2 min heaps for each into one for each interest and these mean heaps will be actually touring the parking spots in an order of the distance from the entrance.
- Apart from that, we will have two sets of parking spots one set is for available parking spots and the other set would be for reserved or booked parking spots. In the beginning, all the parking spots will be available. Suppose we have these two entrances x and y. so we will have actually a map of Min heaps where the key is the Entrance ID and the value is a min-heap where min-heap is a data structure that returns a value that is the smallest of all the values in that data structure. Min heaps are usually implemented in terms of a binary tree where each node has a value that is less than the value of all of its children.
- Whenever you actually want to achieve an object from the min-heap, it will always return you the object which has the minimum value, and min-heap of one parking entrance will have the parking spots will be sorted in the order of the distance from that parking entrance. So when the user calls this getParkingSpot(), it will give us the parking terminal from where it will enter based on the terminal ID we will retrieve the min-heap for that terminal, and then we will just pop the element from the top of the min-heap which will give us a parking spot which is at the minimum distance from that entrance. We will mark that parking spot as reserved. We have to remove it from the set of available parking spot entries.
- This algorithm will have a computational complexity of klog(n)where K is the number of min heaps which is equal to the number of entrances and n is the parking spots’ total count in this parking lot.
Payment Processing
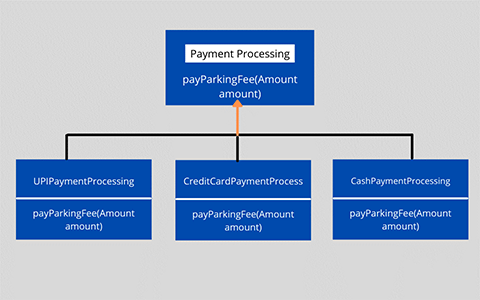
Surveillance System:
we also have a monitoring system that monitoring system can actually use the observer design pattern to actually introduce different components within the system. We can assume that let's suppose our surveillance system has a logger interface that has a function like logMessage().
Parking lot System High Level Designing
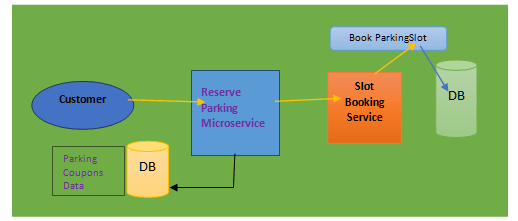
Explanation:
Here in the above diagram, when a customer wants to avail of the parking lot then it tries to book the ticket first and that time the call comes from UI to Reserve parking Service and then from that service to the parking slot service where the parking slot is allocated to the customer and entry time is noted in the DB.
- Let’s construct the parking-lot system on the whole system based on those small components. The first of all the packing law system itself will have a parking lot class and it will be using the singleton design pattern because there will be only one instance of the parking lot system itself.
- This parking lot system which is single to the object will be composed of different smaller objects and I have already mentioned those objects include entry exit terminals parking spots, the assignment strategy etc, the pavement processors. I will be using the factory design pattern to actually instantiate all those objects.
Public APIs:
Internnal Apis:
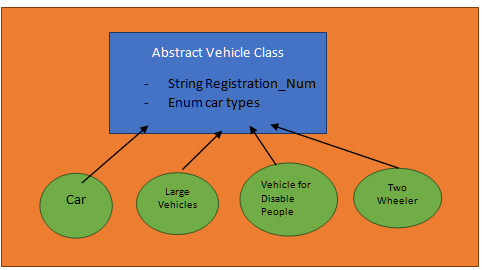
- We have to use multiple lists it could be in the medium list of vehicles or it could also be in place in the small vehicle list if we use instead of four similar lists we use a big one it's going to be the same time complexity. We have to research through one big list and have a linear rate linear time complexity
- Now the implementation of the parking lot system class itself can take a configuration object as input and this config object may have a different values depending on the requirements of the parking lot for example and the number of parking spots, the type of parking spots in it, the payment processor settings etc. And then we can use abstract factory and factory design patterns to instantiate those objects and link them together.
- So, removing a vehicle takes for example that a registration number of the vehicle as to lookup key and the remote vehicle method will just look in Hash Map and that's where we got the spot back and the spot contains the information about the vehicle. That's all we need to place a vehicle and remove the vehicle.
- So, in that case in our all a parking lot system we will have a set of entry and exit terminals that we will actually instantiate. the set of terminus that is the set of entry and exit terminals that will instantiate. Also we will have to instantiate a parking assignment strategy and will pass it a configuration object and from the config object that strategy instance will actually retrieve all the parking spots along with the parking terminals and the distances of the parking spots from each entering terminal etc.
- We have to instantiate the different payment processors and can pass those payment processors to the exit terminals because this is the place where we would be processing the payment.
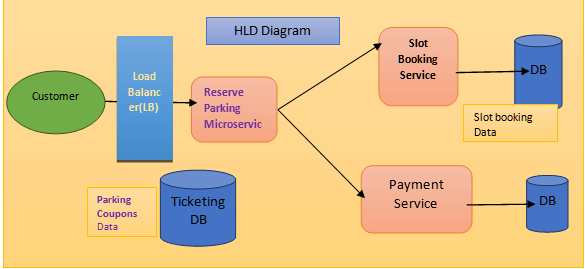
FAQs:
Subject: Parking Lot System Designing:
What are the functional requirements for designing a Parking Lot back-end system?
Ans: Allocation of Parking Spots, Ticket Provisioning to customer, Short distance Parking Sport allocation, Categorizing the Parking Spot, Parking Fee and Parking surveillance system
How to Scale up the requirements in case of multi-level parking slots?
Ans: Increasing number of Parking slots from 5k to 30K, Increase in data Storage due to multiple slots extension,Increasing in data storage due to increase in number of tickets per day(May be per day 1 MB), Maintaining parking lot data for 5 years at least
How to design the back-end system for the Parking Lot requirement?
Ans: Please refer this complete blog to understand the internals of back-end systems for this requirement and also the APIs required to serve this parking lot implementation
What are the public APIs and Private APIs in this parking lot back-end APIs?
Ans: There are couple of public and Private APIs like mainly ParkinglotService API, ParkingSlotType API, SlotService API, PaymentService API
How to find out the nearest parking slot availability?
Ans: The best way to implement it using min heaps. So, we will have a number of min heaps as the number of entrances and all the parking spots will be added to all those min heaps. For each entry terminal there will be a min heap this so we will have 2 min heaps for each into one for each interest and these mean heaps will be actually touring the parking spots in an order of the distance from the entrance.