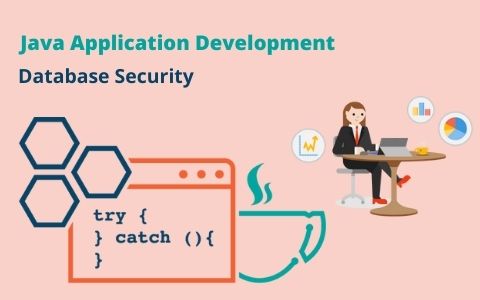
- Perhaps the most obvious way to store a DB password is to simply store a table of usernames and passwords in plain text, but this is a costly bad idea. Any security breach would allow an attacker to see all of your username and password instead your website could try to hide plaintext passwords by encrypting them, but this is still not a safe solution. Encryption requires a key that the server uses to decode the encrypted password and verify user login because of this the key has to be available to the server and can be vulnerable as a result.
- The big question in java application development here is how to keep a hacker from gaining one piece of information that gives them access to every password in your database. The solution is to use a one-way function like hashing. Hashing is a cryptographic technique that takes a plaintext password and creates a random fixed length string of characters this string called a hash is stored by a website instead of plaintext or encrypted password.
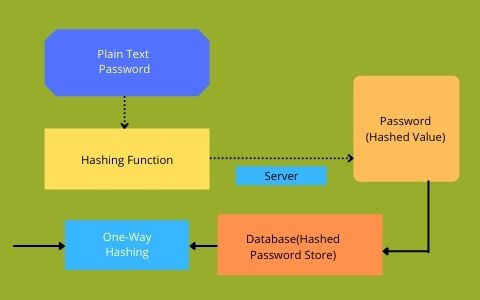
- If this java application development method is implemented when a user tries to log in the website will first hash the password that is entered, it will then retrieve the stored hash for that user. If the entered hash and the stored hash match, then the login is successful. This is significantly more secure than storing the plaintext or encrypted passwords because hash functions have something called preimage resistance which basically means that it's extremely difficult to discover the input based solely on the output of a hash function.
- Even making the smallest change to an input, completely changes the resulting string. In our case, it is nearly impossible to find the actual password which produces a hash stored in our database. So, even if the hacker breaches the database there is no easy way to get back to the original passwords other than just guessing possible inputs into one is found that matches a stored hash.
Concepts of Hashing & Salting the Username/Password
- Now even though hashes are one-way they are still vulnerable to an offline dictionary attack. An attacker can use known hash algorithms and precompute the hash outputs for a dictionary of billions of common passwords. Then should a database be compromised the attacker only needs to compare the stored hashes to their precomputed outputs to break all the accounts with bigger common passwords.
- Additionally, since the specific password will consistently produce the same hash, if users have the same password a hacker will likely be able to compromise both accounts. Since the hashes will also be the same. To prevent this from happening, it is strongly recommended that websites use a method called Salting when storing hash passwords and this involves adding a randomly generated string of characters called the “Salt” to choose individual password before hashing and storing it.
- This added entropy reduces the efficacy of dictionary lookups. While a pre-computer table will contain the hashes for common passwords, it probably won't include those for salted hashes.
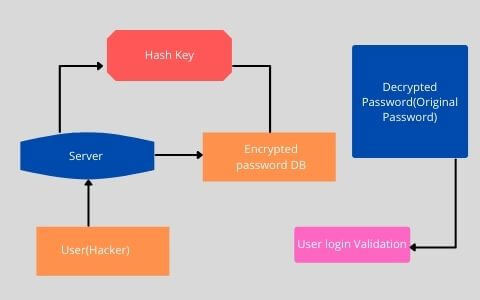
- The salt itself doesn't necessarily have to be secret it just creates a data set of long and random passwords large enough that it makes any sort of dictionary attack infeasible. Salting also resolves the situation in which hacker is able to compromise multiple accounts with the same password using individual randomly generated salts for each user results in unique hashes. So, even if two users have the same password their hashes will look completely different.
- Hashing and salting don't stop database breaches, but they take away what's most important to a hacker time. By making the time cost of each guest extremely high the hackers will be able to break fewer passwords even with a lot of computing power choosing a slower hash. One that takes a long time to run is of great importance it takes too much time to make individual guesses and it will discourage hackers from wasting their resources on your database.
- The question arises here whether reach microservice really need its own database? This question is appropriate for both technical interviews and experienced developers. The key advantage of micro services, which has returned to monolithic, is that it promotes agility by allowing us to build and deploy micro services separately. Each java application development team can work on their own microservices, create them, and deploy them to clients in production.
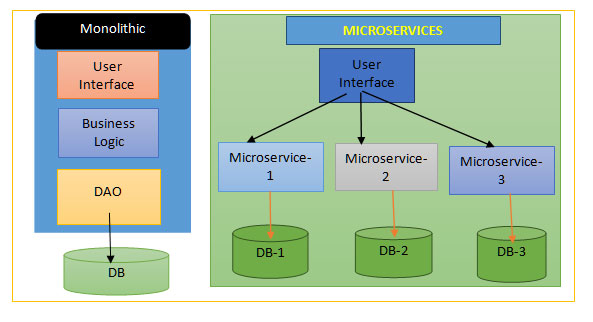
- So, here if we want to achieve this independence, the micro services should be loosely connected to achieve this independence. As a result, each micro service needs to have its own data store. When other micro services require data, the APIs should be used. A database of other services should not be accessed directly by a microservice. It's not a good idea to access a data store that belongs to another microservice directly.
- So now the difficulty now is how to keep the microservice's data storage private. There are various methods for keeping the services database confidential. Private tables per service, schema per service, and database server per service are the main components. For example, we can establish private tables or schemas for each service, and we can create some tables in a database that are only accessible to a specific user.
- It actually depends on the data;for example, if you have a lot of data and want to isolate it completely, you can use a separate database server instance for each microservice. Another issue to consider is that we can have a polyglot persistent architecture. For example, for a text search, we can use elastic search, for a social graph, we can use Neo4j Relational database, and for other micro services, we can use Neo4j Relational database.
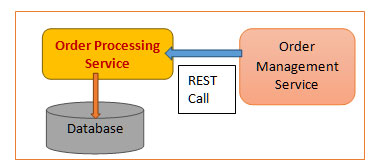
- Here, in this case, one of the benefits of employing micro services is that we can use a variety of databases in our solution. Another difficulty is how to deal with scenarios in which two services want to share data. We'll have to be cautious if two services want to share the same data while I'm modifying the schema because our product development would be delayed
- So, when you’re accessing data, it's always best to use the API. As a result, a single service might be responsible for a set of data while remaining autonomous and loosely connected. Essentially, this is one of the data sharing alternatives.
How To Design Parking Lot Using Java Development Object Oriented Design Pattern
In this blog, you’ll learn about How to Design Parking Lot using Java Object Oriented Design Pattern. You have to concentrate on your system design and especially object-oriented design skills.
Database Authentication in micro services Application:
⮚ Let's imagine we want to construct a user service to store your users to manage authentication. This supports the principles of single responsibility and a single source of point for our data that user service can then support an authenticate end point which your API gateway or your individual micro services can query against.
⮚ Here, we'll want to store and associate permissions to users in the user service to handle authorization. We have two options for handling our authorization business logic. On the one hand, we can use our user service to create an approved endpoint that our micro services can query. You can, on the other hand, delegate authorization business logic to each of your micro services individually. There are a number of trade-offs to be made between these two options.
⮚ So, first thing to understand here is let's start by defining some terminology. Authentication is the process of determining what a user can do in our system once they have been identified. Authorization is the process of determining what a user can do in our system once they have been identified. I've proposed three guiding concepts in analyzing these architecture considerations regarding on-demand and authorization:
⮚ Primary thing and the first point is that the Microservice single responsibility concept, which states that each micro service should be accountable for about one thing in a single domain. The next point is the Single source of truth principle, which states that every piece of information in our microservice system should be authoritatively handled by a single service, and finally, you should consider java application development implementation costs.
⮚ I have mentioned about handling authentication the single responsibility principle and the single source of truth dictate that we should have one source of contact for managing our users or customers. This implies creating a user management service and this service can support an authenticate endpoint. Please refer to the below diagram:
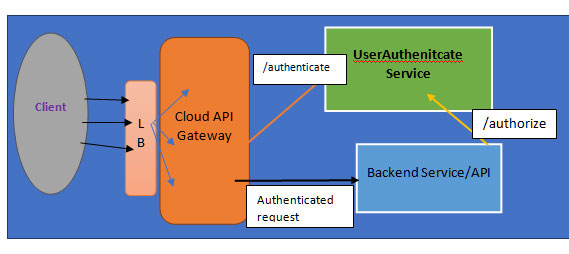
⮚ Let me explain what a Spring Vault is and why we should use it in our micro services applications. Let’s say we have four or more micro services applications that are already deployed to cloud and they are all running fine.
⮚ Let’s assume all those four micro services want to access some common properties for example common secrets (username and password). How we can achieve this? The first solution that we can think of is we can configure those properties value in each and every microservices.
⮚ Actually, the above approach does not look effective. So, the best solution is to centralize the configuration or properties so that each and every micro services can access those value. So, to make this easy Spring developer came up with Spring Cloud Vault concept.
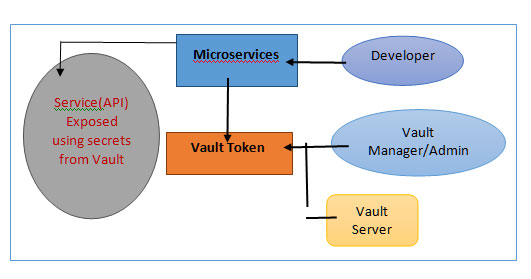
⮚ Basically, Vault will provide some central space where we can configure our secrets like username or password or DB related properties. In the above diagram, in the vault server we can configure username and password, so that all the three or four micro services can directly talk to the Vault to fetch the username and password(Secrets). So, here we do not have to hardcode the common properties or secrets value in each and every microservices.
Vault Implementation in Springboot Microservices:
I have explained the above scenario with a practical example:
⮚ First step is we need to install Vault in our local system. You can visit this site which gives the detailed steps to install Vault. This is spring official site.(https://spring.io/guides/gs/vault-config/). Then you can install and launch Vault link in that page. This page gives installation steps both for Mac and windows users. For windows users, this is the link to download the vault(https://www.vaultproject.io/docs/install).
⮚ After the download just executes the vault.exe file from your local system. You have to configure the vault directory in the system environment variable. Then execute the below command: After running the command you will see the success output as I have given below:


⮚ Now the vault server installed successfully and in this vault server we can be able to write or read or delete the secrets. In this example I have explained how to write the secrets into the vault server from our Springboot application and then I have shown how to read those values as well.
⮚ The next step is we have to set the vault server address through the command:
set VAULT_ADDR = http://127.0.0.1:8200
⮚ Now through the command we will be able to write the secrets into vault server:

⮚ Here spring-vault-demo is my project name. You can give your own project name and aegis.username is the prefix. Password also you can give with prefix like above.
⮚ Now to read that secret there is another command: vault kv get secret/spring-vault-demo
⮚ It will give you the username and password that we have stored in the vault server. You can delete the secrets using the vault kv delete command.
⮚ Now, let’s create a Springboot project. In the project pom file we have to add the spring cloud starter vault maven dependency in the pom file:

⮚ Now, we have to create our main class. We have to create our own POJO for storing the username and password that we have already stored in the vault server.
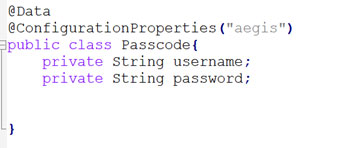
⮚ Then let’s create a VaultConfigApplicationclass where we will be specifying the Passcode class in the enable configuration property annotation. This is our main spring boot application class. I have added constructor injection for the Passcode class here.
⮚ Next is what I have to do in this class is to print the username and password value from the vault server. For that I have to implement the CommandLineRunner interface which will be run at the application startup. Here, I have overridden the run method. Inside the run method I am printing the username and password from vault. Please refer the below image:
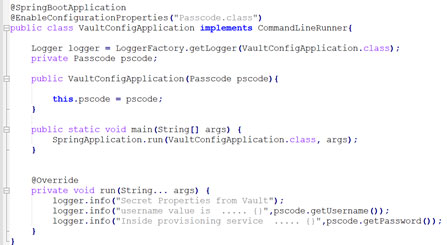
⮚ Just remember that the above vault configuration class always reads the bootstrap.properties file from resource folder. So, we need to create a new file named “bootstrap.properties” in our project’s resource folder. In this property file, we have to mention the application name which in our case is spring-vault-demo.
⮚ The next field is vault token you need to specify in this file. This is the same token with which we started our vault server. The next is the scheme filed which is “http” as our vault address is in http mode. The last one is the kv enabled flag which should be set as true.

⮚ Now if you run your Springboot application then you can be able to see the username and password field printed. Then you can delete the secret values from vault server using delete command and then again try to rerun the Springboot java application development. That time it will print null values as there is no data in the vault.
FAQs:
Subject: Parking Lot System Designing:
Why do we need Database Security?
Ans: To store a DB password is to simply store a table of usernames and passwords in plain text, but this is a costly bad idea. Any security breach would allow an attacker to see all of your username and password instead your website could try to hide plaintext passwords by encrypting them
sWhat is Hashing and Salting in Password Security?
Ans: Salting is when storing hash passwords and this involves adding a randomly generated string of characters called the “Salt” to choose an individual password before hashing and storing it. The specific user password will consistently produce the same hash, if users have the same password a hacker will likely be able to compromise both accounts.
What is Vault and why it is more popular now days?
Ans: Vault will provide some central space where we can configure our secrets like username or password or DB related properties. In the above diagram, in the vault server we can configure username and password, so that all the micro services can directly talk to the Vault to fetch the username and password (Secrets).
How to implement Vault with Springboot micro services Application?
Ans: Spring provides vault support and the complete example I have explained in this blog. Please refer the detailed steps to implement vault in microservices.
What are the best practices to follow to maintain application and DB Security in Java based micro services Applications?
Ans: There should not be multiple DB configured in Each of the Microservices. Handling authentication the single responsibility principle and the single source of truth dictate that we should have one source of contact for managing our users or customers. The best solution is to centralize the configuration or properties so that each and every micro services can access those value. So, to make this easy Spring developer came up with Spring Cloud Vault concept.